# Simple calculator program# Function to add two numbersdef add(x, y):return x + y# Function to subtract two numbersdef subtract(x, y):return x - y# Function to multiply two numbersdef multiply(x, y):return x * y# Function to divide two numbersdef divide(x, y):return x / y# Display menuprint("Select operation.")print("1. Add")print("2. Subtract")print("3. Multiply")print("4. Divide")# Take input from the userchoice =input("Enter choice (1/2/3/4): ")num1 =float(input("Enter first number: "))num2 =float(input("Enter second number: "))# Perform operation based on user's choiceif choice =='1':print(num1, "+", num2, "=", add(num1, num2))elif choice =='2':print(num1, "-", num2, "=", subtract(num1, num2))elif choice =='3':print(num1, "*", num2, "=", multiply(num1, num2))elif choice =='4':print(num1, "/", num2, "=", divide(num1, num2))else:print("Invalid input")
how to run this python code
Python 코드를 실행하려면 시스템에 Python이 설치되어 있어야 합니다. 다음 단계에 따라 간단한 계산기를 위한 Python 코드를 실행할 수 있습니다:
메모장이나 서브라인 텍스트와 같은 텍스트 편집기를 열고 Python 코드를 새 파일에 붙여넣습니다.
파일 확장명을 .py로 지정하여 저장합니다(예: calculator.py).
터미널 또는 명령 프롬프트 창을 엽니다.
cd 명령을 사용하여 파일이 저장된 디렉토리로 이동합니다(예: cd Documents\Python).
python calculator.py를 입력하고 Enter 키를 눌러 프로그램을 실행합니다.
그러면 프로그램이 실행되고 메뉴가 표시되며 사용자에게 원하는 연산과 숫자 두 개를 입력하라는 메시지가 표시됩니다. 사용자가 필요한 입력을 입력하면 프로그램이 해당 산술 연산을 수행하고 결과를 표시합니다.
코드
xfun::embed_file("code/calculator.py", text ="계산기 소스코드 다운로드")
이번에는 계산기에 GUI를 입혀 사용자가 좀더 쉽게 사용할 수 있도록 한다. 다양한 GUI 패키지가 있지만 그중에서 tkinter 패키지 하나를 선정하여 개발을 이어나간다. chatGPT에 지시명령어로 계산기 프로그램을 파이썬 언어로 작성하라는 하명을 내린다. 계산기를 동작시키는 방식도 알려달라는 하명도 이어서 내린다.
Sure, here is an example of the same calculator program using the Tkinter library to create a graphical user interface (GUI):
…
In this code, we first import the Tkinter library using the alias tk. Then we define four functions add(), subtract(), multiply(), and divide() that perform the corresponding arithmetic operations on the numbers entered by the user. Each function retrieves the input values from the Entry widgets using the get() method, performs the arithmetic operation, and updates the text of the Label widget to display the result.
We then create the main window using the Tk() constructor and set its title using the title() method. Next, we create two Label widgets and two Entry widgets to allow the user to enter the two numbers.
We also create four Button widgets, one for each arithmetic operation, and set their text and width. The command parameter is used to specify the function that should be called when the button is clicked.
Finally, we create a Label widget to display the result of the arithmetic operation, and call the mainloop() method to start the event loop and display the GUI.
코드
import tkinter as tk# Function to perform additiondef add():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 + num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")# Function to perform subtractiondef subtract():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 - num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")# Function to perform multiplicationdef multiply():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 * num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")# Function to perform divisiondef divide():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 / num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")exceptZeroDivisionError: label_result.config(text="Cannot divide by zero")# Create the main windowwindow = tk.Tk()window.title("Simple Calculator")# Create the number input fieldslabel_num1 = tk.Label(window, text="Number 1:")label_num1.pack()entry_num1 = tk.Entry(window)entry_num1.pack()label_num2 = tk.Label(window, text="Number 2:")label_num2.pack()entry_num2 = tk.Entry(window)entry_num2.pack()# Create the operation buttonsbutton_add = tk.Button(window, text="+", width=10, command=add)button_add.pack()button_subtract = tk.Button(window, text="-", width=10, command=subtract)button_subtract.pack()button_multiply = tk.Button(window, text="*", width=10, command=multiply)button_multiply.pack()button_divide = tk.Button(window, text="/", width=10, command=divide)button_divide.pack()# Create the result labellabel_result = tk.Label(window, text="Result: ")label_result.pack()# Run the main loopwindow.mainloop()
how to run this python code
Python 코드를 실행하려면 시스템에 Python이 설치되어 있어야 합니다. 다음 단계에 따라 간단한 계산기를 위한 Python 코드를 실행할 수 있습니다:
메모장이나 서브라인 텍스트와 같은 텍스트 편집기를 열고 Python 코드를 새 파일에 붙여넣습니다.
파일 확장명을 .py로 지정하여 저장합니다(예: calculator.py).
터미널 또는 명령 프롬프트 창을 엽니다.
cd 명령을 사용하여 파일이 저장된 디렉토리로 이동합니다(예: cd Documents\Python).
python calculator.py를 입력하고 Enter 키를 눌러 프로그램을 실행합니다.
그러면 프로그램이 실행되고 메뉴가 표시되며 사용자에게 원하는 연산과 숫자 두 개를 입력하라는 메시지가 표시됩니다. 사용자가 필요한 입력을 입력하면 프로그램이 해당 산술 연산을 수행하고 결과를 표시합니다.
코드
xfun::embed_file("code/calculator_gui.py", text ="계산기 소스코드 GUI 버전 다운로드")
first write python program for calculator and then test result from execution
Here’s the Python code for a simple calculator that supports addition, subtraction, multiplication, and division. We’ll create a separate file for the test cases, which will import and run the calculator functions.
Save the following code as calculator.py:
class Calculator:def add(self, a, b):return a + bdef subtract(self, a, b):return a - bdef multiply(self, a, b):return a * bdef divide(self, a, b):if b ==0:raiseValueError("Cannot divide by zero")return a / b
Now, create a new file named test_calculator.py and save the following code in it:
from calculator import Calculatordef test_calculator(): calc = Calculator()# Test additionassert calc.add(2, 3) ==5, "Test case 1 failed"assert calc.add(-2, 3) ==1, "Test case 2 failed"# Test subtractionassert calc.subtract(5, 3) ==2, "Test case 3 failed"assert calc.subtract(0, -5) ==5, "Test case 4 failed"# Test multiplicationassert calc.multiply(3, 4) ==12, "Test case 5 failed"assert calc.multiply(-2, 5) ==-10, "Test case 6 failed"# Test divisionassert calc.divide(10, 2) ==5, "Test case 7 failed"assert calc.divide(-10, 5) ==-2, "Test case 8 failed"# Test division by zerotry: calc.divide(10, 0)exceptValueErroras e:assertstr(e) =="Cannot divide by zero", "Test case 9 failed"print("All test cases passed")if__name__=="__main__": test_calculator()
To execute the test cases, open a terminal or command prompt and navigate to the directory containing both calculator.py and test_calculator.py. Then run the following command:
python test_calculator.py
If all test cases pass, you’ll see “All test cases passed” printed on the console.
4.exe 배포
CLI를 통해 기능을 검증한 후 GUI로 사용자가 사용하기 쉬운 인터페이스를 구현하였다면 다음 단계로 배포를 한다. 다양한 배포 프로그램이 존재하지만 가장 쉽게 .exe 파일을 만드는 방법을 살펴보자.
I am a pyhton developer. I have a simple python calculator. I’d like to deploy this program into Windows. let me know how to create a standalone executable file
To create a standalone executable file for your Python calculator, you can use a tool like PyInstaller or cx_Freeze. Here are the general steps to follow:
Install PyInstaller: You can use pip to install PyInstaller. Open a command prompt or terminal and run the following command:
pip install pyinstaller
Create a spec file: PyInstaller requires a spec file that describes your Python program and its dependencies. To create a spec file with PyInstaller, run the following command:
Build the executable: To build the executable file with PyInstaller, run the following command:
pyinstaller calculator.spec
This will create a standalone executable file in the dist directory. Test the executable file: Run the executable file on a Windows machine to make sure it works as expected.
By following these steps, you can create a standalone executable file for your Python calculator using PyInstaller.
---title: "chatGPT"subtitle: "계산기 프로그램"author: - name: 이광춘 url: https://www.linkedin.com/in/kwangchunlee/ affiliation: 한국 R 사용자회 affiliation-url: https://github.com/bit2rtitle-block-banner: true#title-block-banner: "#562457"format: html: css: css/quarto.css theme: flatly code-fold: true code-overflow: wrap toc: true toc-depth: 3 toc-title: 목차 number-sections: true highlight-style: github self-contained: falsefilters: - lightbox - custom-callout.lua lightbox: autolink-citations: yesknitr: opts_chunk: message: false warning: false collapse: true comment: "#>" R.options: knitr.graphics.auto_pdf: trueeditor_options: chunk_output_type: console---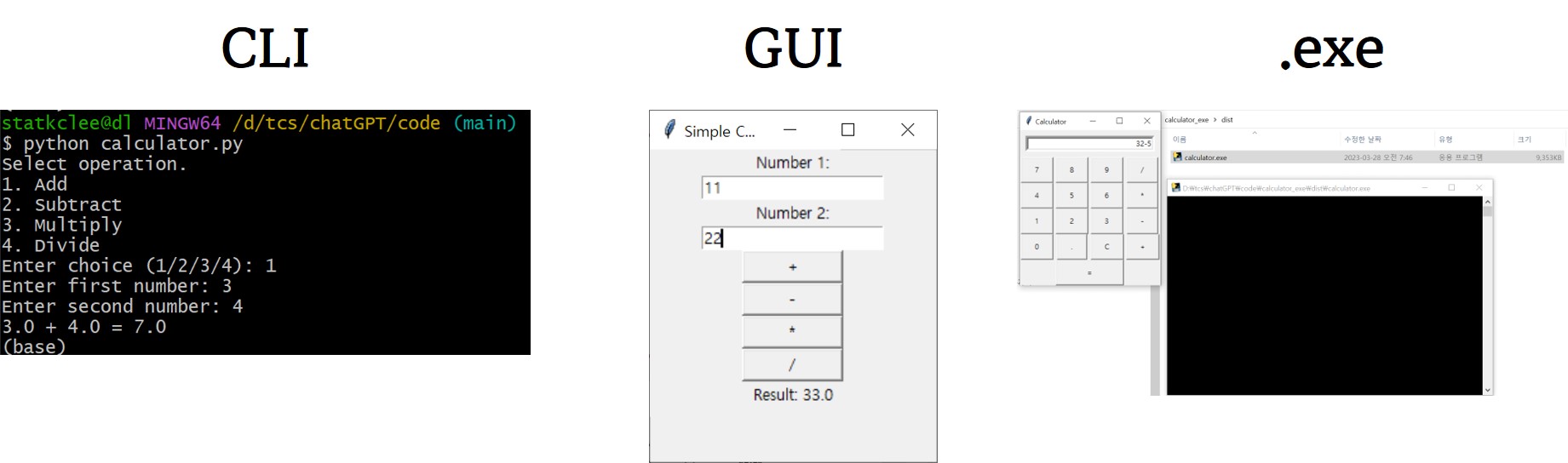# CLI 계산기chatGPT에 지시명령어로 계산기 프로그램을 파이썬 언어로 작성하라는 하명을 내린다.계산기를 동작시키는 방식도 알려달라는 하명도 이어서 내린다.::: panel-tabset## 지시명령어::: {.callout-note collapse="true"}### write python code of calculator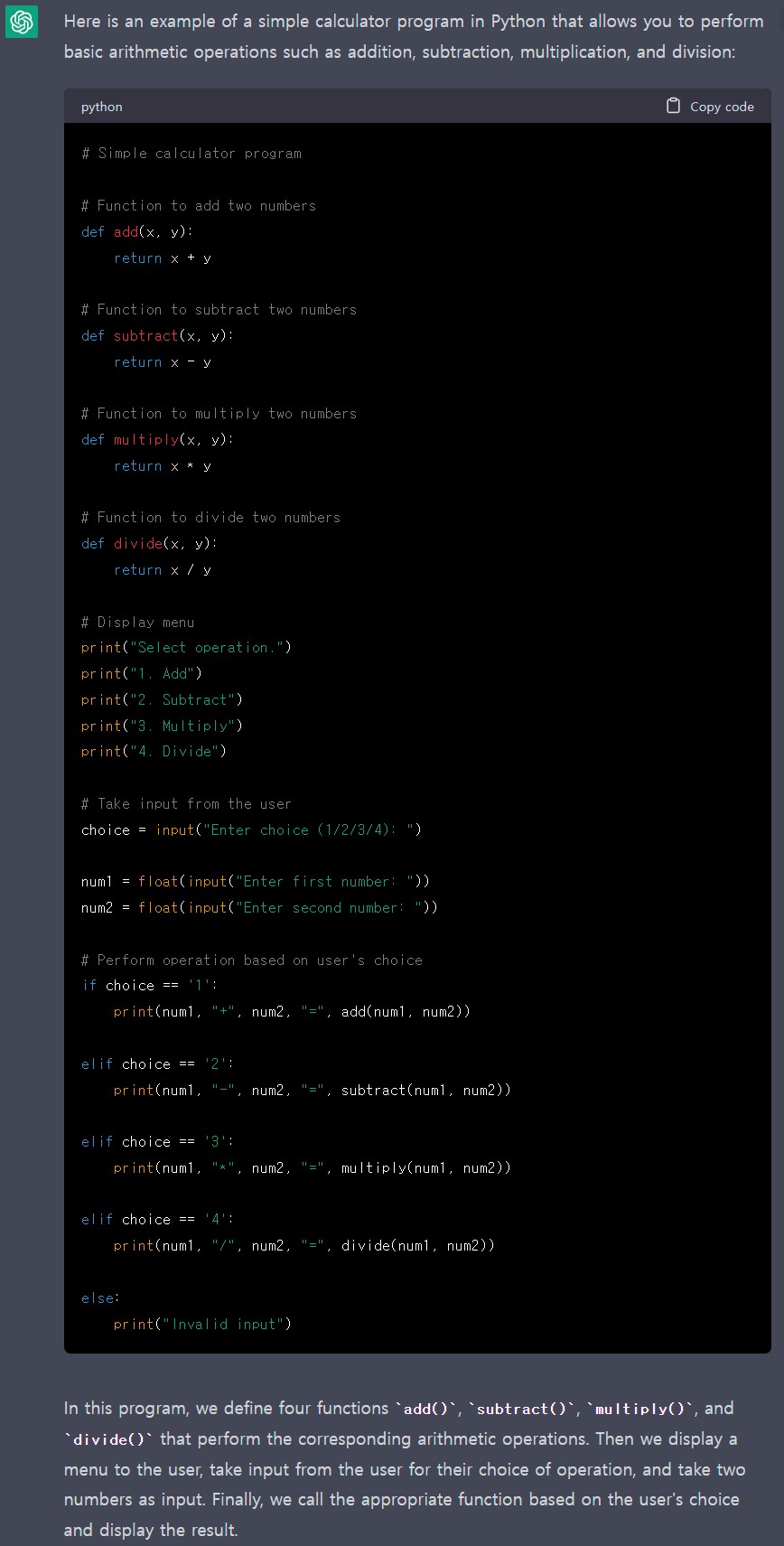:::## 소스코드```{python}#| eval: false# Simple calculator program# Function to add two numbersdef add(x, y):return x + y# Function to subtract two numbersdef subtract(x, y):return x - y# Function to multiply two numbersdef multiply(x, y):return x * y# Function to divide two numbersdef divide(x, y):return x / y# Display menuprint("Select operation.")print("1. Add")print("2. Subtract")print("3. Multiply")print("4. Divide")# Take input from the userchoice =input("Enter choice (1/2/3/4): ")num1 =float(input("Enter first number: "))num2 =float(input("Enter second number: "))# Perform operation based on user's choiceif choice =='1':print(num1, "+", num2, "=", add(num1, num2))elif choice =='2':print(num1, "-", num2, "=", subtract(num1, num2))elif choice =='3':print(num1, "*", num2, "=", multiply(num1, num2))elif choice =='4':print(num1, "/", num2, "=", divide(num1, num2))else:print("Invalid input")```## →## 실행 지시명령어::: {.callout-note collapse="false"}### how to run this python codePython 코드를 실행하려면 시스템에 Python이 설치되어 있어야 합니다. 다음 단계에 따라 간단한 계산기를 위한 Python 코드를 실행할 수 있습니다:1. 메모장이나 서브라인 텍스트와 같은 텍스트 편집기를 열고 Python 코드를 새 파일에 붙여넣습니다.1. 파일 확장명을 .py로 지정하여 저장합니다(예: calculator.py).1. 터미널 또는 명령 프롬프트 창을 엽니다.1. `cd` 명령을 사용하여 파일이 저장된 디렉토리로 이동합니다(예: `cd Documents\Python`).1. `python calculator.py`를 입력하고 Enter 키를 눌러 프로그램을 실행합니다.그러면 프로그램이 실행되고 메뉴가 표시되며 사용자에게 원하는 연산과 숫자 두 개를 입력하라는 메시지가 표시됩니다. 사용자가 필요한 입력을 입력하면 프로그램이 해당 산술 연산을 수행하고 결과를 표시합니다.:::## 실행 사례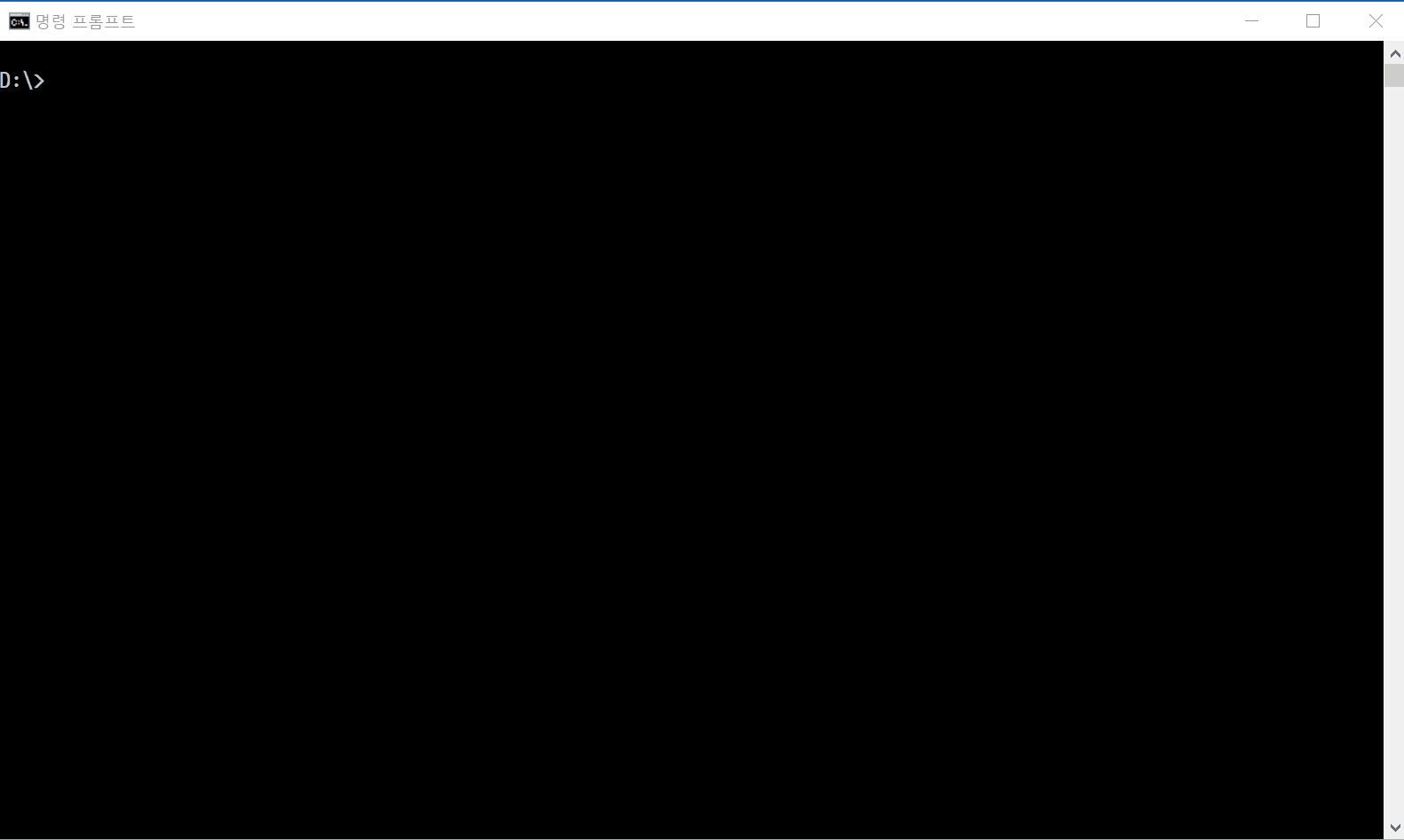:::```{r}xfun::embed_file("code/calculator.py", text ="계산기 소스코드 다운로드")```# GUI 계산기이번에는 계산기에 GUI를 입혀 사용자가 좀더 쉽게 사용할 수 있도록 한다.다양한 GUI 패키지가 있지만 그중에서 `tkinter` 패키지 하나를 선정하여 개발을 이어나간다.chatGPT에 지시명령어로 계산기 프로그램을 파이썬 언어로 작성하라는 하명을 내린다.계산기를 동작시키는 방식도 알려달라는 하명도 이어서 내린다.::: panel-tabset## 지시명령어::: {.callout-note collapse="true"}### please add gui with tkinter Sure, here is an example of the same calculator program using the Tkinter library to create a graphical user interface (GUI):...In this code, we first import the Tkinter library using the alias tk. Then we define four functions add(), subtract(), multiply(), and divide() that perform the corresponding arithmetic operations on the numbers entered by the user. Each function retrieves the input values from the Entry widgets using the get() method, performs the arithmetic operation, and updates the text of the Label widget to display the result.We then create the main window using the Tk() constructor and set its title using the title() method. Next, we create two Label widgets and two Entry widgets to allow the user to enter the two numbers.We also create four Button widgets, one for each arithmetic operation, and set their text and width. The command parameter is used to specify the function that should be called when the button is clicked.Finally, we create a Label widget to display the result of the arithmetic operation, and call the mainloop() method to start the event loop and display the GUI.:::## 소스코드```{python}#| eval: falseimport tkinter as tk# Function to perform additiondef add():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 + num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")# Function to perform subtractiondef subtract():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 - num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")# Function to perform multiplicationdef multiply():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 * num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")# Function to perform divisiondef divide():try: num1 =float(entry_num1.get()) num2 =float(entry_num2.get()) result = num1 / num2 label_result.config(text="Result: "+str(result))exceptValueError: label_result.config(text="Invalid input")exceptZeroDivisionError: label_result.config(text="Cannot divide by zero")# Create the main windowwindow = tk.Tk()window.title("Simple Calculator")# Create the number input fieldslabel_num1 = tk.Label(window, text="Number 1:")label_num1.pack()entry_num1 = tk.Entry(window)entry_num1.pack()label_num2 = tk.Label(window, text="Number 2:")label_num2.pack()entry_num2 = tk.Entry(window)entry_num2.pack()# Create the operation buttonsbutton_add = tk.Button(window, text="+", width=10, command=add)button_add.pack()button_subtract = tk.Button(window, text="-", width=10, command=subtract)button_subtract.pack()button_multiply = tk.Button(window, text="*", width=10, command=multiply)button_multiply.pack()button_divide = tk.Button(window, text="/", width=10, command=divide)button_divide.pack()# Create the result labellabel_result = tk.Label(window, text="Result: ")label_result.pack()# Run the main loopwindow.mainloop()```## →## 실행 지시명령어::: {.callout-note collapse="false"}### how to run this python codePython 코드를 실행하려면 시스템에 Python이 설치되어 있어야 합니다. 다음 단계에 따라 간단한 계산기를 위한 Python 코드를 실행할 수 있습니다:1. 메모장이나 서브라인 텍스트와 같은 텍스트 편집기를 열고 Python 코드를 새 파일에 붙여넣습니다.1. 파일 확장명을 .py로 지정하여 저장합니다(예: calculator.py).1. 터미널 또는 명령 프롬프트 창을 엽니다.1. `cd` 명령을 사용하여 파일이 저장된 디렉토리로 이동합니다(예: `cd Documents\Python`).1. `python calculator.py`를 입력하고 Enter 키를 눌러 프로그램을 실행합니다.그러면 프로그램이 실행되고 메뉴가 표시되며 사용자에게 원하는 연산과 숫자 두 개를 입력하라는 메시지가 표시됩니다. 사용자가 필요한 입력을 입력하면 프로그램이 해당 산술 연산을 수행하고 결과를 표시합니다.:::## 실행 사례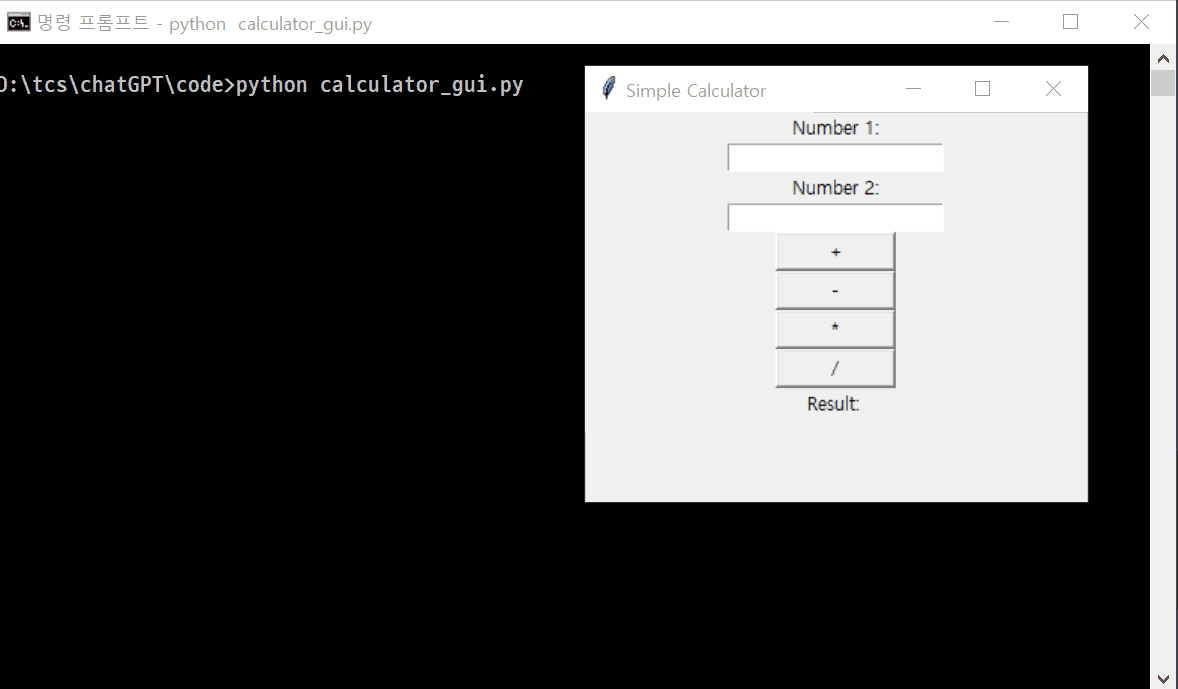:::```{r}xfun::embed_file("code/calculator_gui.py", text ="계산기 소스코드 GUI 버전 다운로드")```# 테스트계산기 프로그램이 정상동작하는지 확인하는 코드를 작성하자.만약 단위 테스트 결과가 모두 정상동작한다면 믿고 현업에서 사용하게 된다.::: panel-tabset## 지시명령어::: {.callout-note collapse="true"}### first write python program for calculator and then test result from executionHere's the Python code for a simple calculator that supports addition, subtraction, multiplication, and division. We'll create a separate file for the test cases, which will import and run the calculator functions.Save the following code as calculator.py:```pythonclass Calculator:def add(self, a, b):return a + bdef subtract(self, a, b):return a - bdef multiply(self, a, b):return a * bdef divide(self, a, b):if b ==0:raiseValueError("Cannot divide by zero")return a / b```Now, create a new file named test_calculator.py and save the following code in it:```pythonfrom calculator import Calculatordef test_calculator(): calc = Calculator()# Test additionassert calc.add(2, 3) ==5, "Test case 1 failed"assert calc.add(-2, 3) ==1, "Test case 2 failed"# Test subtractionassert calc.subtract(5, 3) ==2, "Test case 3 failed"assert calc.subtract(0, -5) ==5, "Test case 4 failed"# Test multiplicationassert calc.multiply(3, 4) ==12, "Test case 5 failed"assert calc.multiply(-2, 5) ==-10, "Test case 6 failed"# Test divisionassert calc.divide(10, 2) ==5, "Test case 7 failed"assert calc.divide(-10, 5) ==-2, "Test case 8 failed"# Test division by zerotry: calc.divide(10, 0)exceptValueErroras e:assertstr(e) =="Cannot divide by zero", "Test case 9 failed"print("All test cases passed")if__name__=="__main__": test_calculator()```To execute the test cases, open a terminal or command prompt and navigate to the directory containing both calculator.py and test_calculator.py. Then run the following command:```bashpython test_calculator.py```If all test cases pass, you'll see "All test cases passed" printed on the console.:::## 실행결과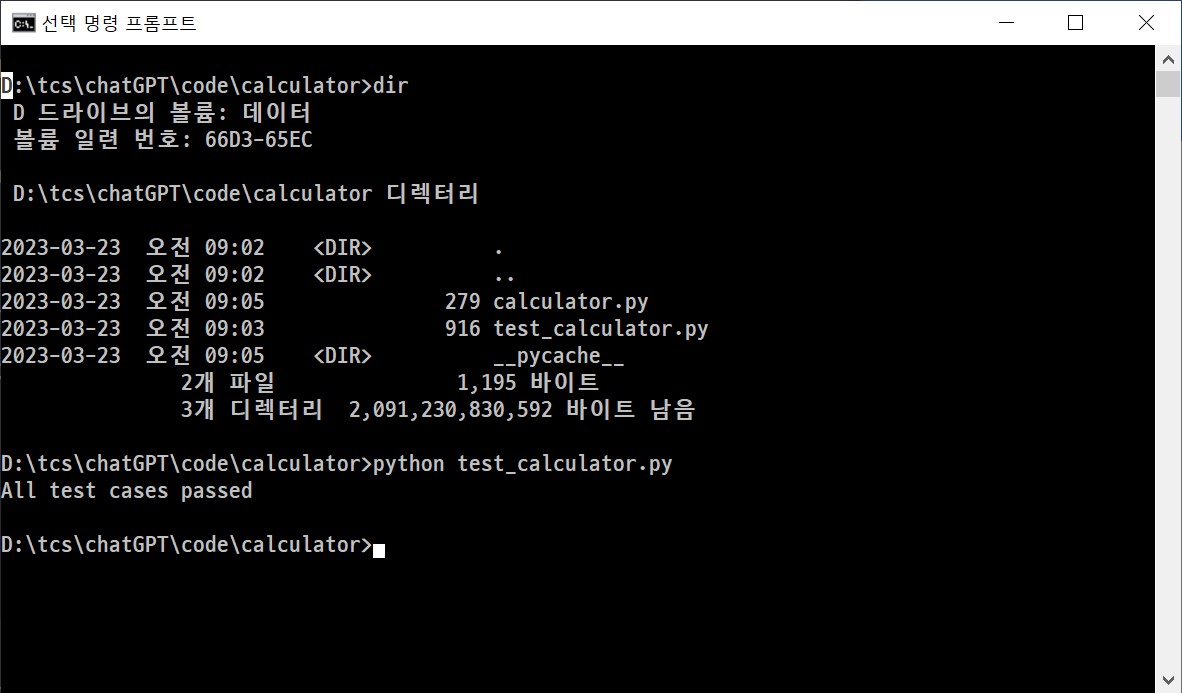:::# `.exe` 배포CLI를 통해 기능을 검증한 후 GUI로 사용자가 사용하기 쉬운 인터페이스를 구현하였다면 다음 단계로 배포를 한다. 다양한 배포 프로그램이 존재하지만 가장 쉽게 `.exe` 파일을 만드는 방법을 살펴보자.::: panel-tabset## 지시명령어::: {.callout-note collapse="true"}### I am a pyhton developer. I have a simple python calculator. I'd like to deploy this program into Windows. **let me know how to create a standalone executable file**To create a standalone executable file for your Python calculator, you can use a tool like PyInstaller or cx_Freeze. Here are the general steps to follow:Install PyInstaller: You can use pip to install PyInstaller. Open a command prompt or terminal and run the following command:```pip install pyinstaller```Create a spec file: PyInstaller requires a spec file that describes your Python program and its dependencies. To create a spec file with PyInstaller, run the following command:```pyinstaller --name=calculator --onefile calculator.py```Build the executable: To build the executable file with PyInstaller, run the following command:```pyinstaller calculator.spec```This will create a standalone executable file in the dist directory.Test the executable file: Run the executable file on a Windows machine to make sure it works as expected.By following these steps, you can create a standalone executable file for your Python calculator using PyInstaller.:::## 실행결과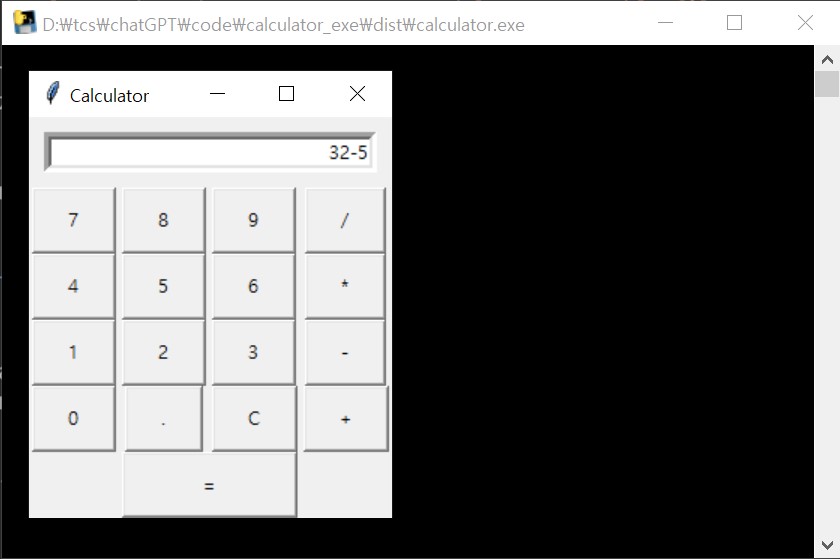## 파이썬 코드```{python}#| eval: falseimport tkinter as tkclass Calculator:def__init__(self, master):self.master = masterself.master.title("Calculator")# Create displayself.display = tk.Entry(self.master, width=30, borderwidth=5, justify=tk.RIGHT)self.display.grid(row=0, column=0, columnspan=4, padx=10, pady=10)# Define buttons button_list = ["7", "8", "9", "/", "4", "5", "6", "*", "1", "2", "3", "-", "0", ".", "C", "+"]# Create buttons row =1 col =0for button in button_list: command =lambda x=button: self.button_click(x) tk.Button(self.master, text=button, padx=20, pady=10, command=command).grid(row=row, column=col) col +=1if col >3: row +=1 col =0# Create equals button tk.Button(self.master, text="=", padx=50, pady=10, command=self.calculate).grid(row=row, column=0, columnspan=4)def button_click(self, button):if button =="C":self.display.delete(0, tk.END)else:self.display.insert(tk.END, button)def calculate(self):try: result =eval(self.display.get())self.display.delete(0, tk.END)self.display.insert(0, result)except:self.display.delete(0, tk.END)self.display.insert(0, "Error")# Create windowroot = tk.Tk()# Create calculatorcalculator = Calculator(root)# Run windowroot.mainloop()```## `.spec` 파일```{python}#| eval: false# -*- mode: python ; coding: utf-8 -*-block_cipher =Nonea = Analysis( ['calculator.py'], pathex=[], binaries=[], datas=[], hiddenimports=[], hookspath=[], hooksconfig={}, runtime_hooks=[], excludes=[], win_no_prefer_redirects=False, win_private_assemblies=False, cipher=block_cipher, noarchive=False,)pyz = PYZ(a.pure, a.zipped_data, cipher=block_cipher)exe = EXE( pyz, a.scripts, a.binaries, a.zipfiles, a.datas, [], name='calculator', debug=False, bootloader_ignore_signals=False, strip=False, upx=True, upx_exclude=[], runtime_tmpdir=None, console=True, disable_windowed_traceback=False, argv_emulation=False, target_arch=None, codesign_identity=None, entitlements_file=None,)```## 디렉토리 구조```{r}fs::dir_tree('code/calculator_exe/')```:::